Chuyển đổi tiền tệ với API miễn phí – Sử dụng VueJS kết hợp Laravel
Bạn đã từng sử dụng API miễn phí cho việc chuyển đổi các đơn vị tiền tệ chưa, hãy cùng theo dõi ví dụ dưới đây nhé.
Currency-exchange trong rapidapi cung cấp bản miễn phí cho chúng ta 2 API, đó là: List các đơn vị tiền tệ và Chuyển đổi tiền tệ.
Để sử dụng API này các bạn có thể vào đăng ký miễn phí một tài khoản tại rapidapi.com, bạn sẽ lấy được một API Key như sau: (4929d1ffc6mshdd7c3bea2cb844dp1e83xxxxxxxxxxx). Chúng ta chỉ cần API Key này và bắt đầu thôi.
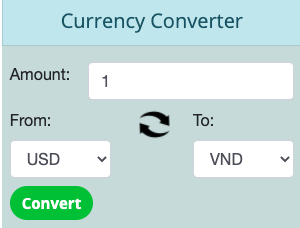
Chuyển đổi tiền tệ với API miễn phí
1. File Api route
Gọi tới hai hàm được xây dựng trong một controller CurrencyController:
– currencyListquotes: Danh sách đơn vị tiền tệ
– currencyConverter: Chuyển đổi các đơn vị tiền tệ
Route::get(‘/currencyListquotes’, ‘Currency\CurrencyController@currencyListquotes’);
Route::get(‘/currencyConverter/{currency_from}/{currency_to}’, ‘Currency\CurrencyController@currencyConverter’);
2. Controller
Bạn tạo một controller CurrencyController Api, xây dựng hai hàm lấy danh sách tiền tệ và chuyển đổi tiền tệ như dưới đây, chúng ta sử dụng curl của php để lấy kết quả trả về dạng JSON.
namespace App\Http\Controllers\Api\Currency;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;class CurrencyController extends Controller
{
public function currencyListquotes()
{
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => “https://currency-exchange.p.rapidapi.com/listquotes”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “GET”,
CURLOPT_HTTPHEADER => array(
“x-rapidapi-host: currency-exchange.p.rapidapi.com”,
“x-rapidapi-key: 4929d1ffc6mshdd7c3bea2cb844dp1e83xxxxxxxxxxx” // API Key của bạn
),
));$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);return $response;
}public function currencyConverter($currency_from, $currency_to)
{
$curl = curl_init();curl_setopt_array($curl, array(
CURLOPT_URL => “https://currency-exchange.p.rapidapi.com/exchange?q=1.0&from=$currency_from&to=$currency_to”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “GET”,
CURLOPT_HTTPHEADER => array(
“x-rapidapi-host: currency-exchange.p.rapidapi.com”,
“x-rapidapi-key: 4929d1ffc6mshdd7c3bea2cb844dp1e83xxxxxxxxxxx” // API Key của bạn
),
));$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);return $response;
}
}
3. File VueJS
Sau khi đã có kết quả trả về từ Controller, việc tiếp theo là chúng ta xây dựng FrontEnd để show kết quả, File VueJS currency.vue trong đường dẫn /resources/assets/js/views sẽ có nội dung như sau:
<div class=”list-group text-left mb-2″>
<h4 class=”list-group-item list-group-item-action list-group-item-info mb-2 text-center”>Currency Converter</h4>
<div class=”form-group m-2″>
<div class=”row”>
<div class=”col-md-12 mb-2″>
<div class=”row”>
<label for=”amount” class=”float-left text-left col-md-3″>Amount:</label>
<div class=”col-md-9″>
<input type=”number” class=”form-control” id=”amount” v-model=”currency_amount”>
</div>
</div>
</div>
<div class=”col-md-5″>
<label for=”currency_from” class=”float-left”>From:</label>
<select class=”form-control currency_from” v-model=”currency_from_default” id=”currency_from”>
<option v-for=”index in currencyListquotes” v-bind:value=”index”>{{index}}</option>
</select>
</div><div class=”col-md-2 text-center pl-0 pr-0″ style=””>
<label></label>
<img :src=”‘https://popularinfo.net/images/arrow-exchange.png'” alt=”exchange” width=”33px” @click=”changePosition”/>
</div><div class=”col-md-5″>
<label for=”currency_to” class=”float-left”>To:</label>
<select class=”form-control currency_to” v-model=”currency_to_default” id=”currency_to”>
<option v-for=”index in currencyListquotes” v-bind:value=”index”>{{index}}</option>
</select>
</div>
<div class=”col-md-12 mt-2 mb-2″>
<button type=”button” class=”btn btn-success float-left” @click=”getCurrencyChange” style=”font-size: 15px;text-transform: inherit;”>Convert</button>
<span v-if=”currencyResult” class=”text-right float-right font-weight-bold”>
<span v-if=”loading”>
<img :src=”‘/images/loading-icon2.gif'” alt=”loading” width=”25px”/>
</span>
<span v-if=”!loading”>
{{ currencyResult }}
</span>
</span>
</div>
</div>
</div>
</div>
– Đặt mặc định đơn vị chuyển đổi là 1 đơn vị từ USD sang VND
– Đổi ngược lại 2 đơn vị tiền tệ với nút exchange ở giữa
– axios: Hàm này sử dụng get API
– helper: File chứa hàm định dạng lại chuỗi kết quả tiền tệ.
<script>
import axios from ‘axios’;
import helper from ‘helper’;export default {
data () {
return {
currencyResult: ”, currencyListquotes: ”, currency_from_default: ‘USD’, currency_to_default: ‘VND’,
currency_amount: ‘1’,
loading: false,
}
},created() {
this.listCurrency();
},methods: {
// Lấy danh sách đơn vị tiền tệ
listCurrency: function () {
axios
.get(‘/api/currencyListquotes’)
.then(response => {
this.currencyListquotes = response.data;}).catch(error => {
});
},// Đổi ngược lại 2 đơn vị tiền tệ
changePosition: function () {
let from = document.getElementById(“currency_from”);
let currency_from = from.options[from.selectedIndex].text;let to = document.getElementById(“currency_to”);
let currency_to = to.options[to.selectedIndex].text;from.value = currency_to;
to.value = currency_from;
},// Truyền lên API chuyển đổi với tham số là số tiền, đơn vị tiền tệ
getCurrencyChange: function () {
this.loading = true;let amount = document.getElementById(“amount”).value;
if (!amount || amount == 0) { amount = 1; }let from = document.getElementById(“currency_from”);
let currency_from = from.options[from.selectedIndex].text;let to = document.getElementById(“currency_to”);
let currency_to = to.options[to.selectedIndex].text;this.getCurrencyConverter(amount, currency_from, currency_to);
},// Lấy kết quả chuyển đổi
getCurrencyConverter(amount, currency_from, currency_to) {
this.loading = true;
axios
.get(‘/api/currencyConverter/’ + currency_from + ‘/’ + currency_to)
.then(response => {
let data = response.data;if (data) {
data = amount * data;
this.currencyResult = helper.numberWithCommas(data) + ‘ ‘ + currency_to;
} else {
this.currencyResult = data;
}
this.loading = false;
}).catch(error => {});
},
}
}
</script>
4. File helper.js
Hàm định dạng lại chuỗi thập phân
// Ví dụ:
// 12.34546 -> 12.345
// 42345255.356 -> 42,345,255.356export default {
numberWithCommas: function(x) {
return (x).toFixed(3).replace(/\d(?=(\d{3})+\.)/g, ‘$&,’)
},
}
Bình luận